Clean Architecture: Implementation Using Quarkus + Gradle Multi-Project. Part 4
Welcome to the fourth installment of our journey into Clean Architecture with Quarkus and Java 17.
In this chapter, we're diving deep into the configuration of the
infrastructure/data
module.
Configuring Infrastructure Data Module: Hibernate Reactive + Panache + MySQL
Welcome back to our exploration of Clean Architecture with Quarkus and Java 17. In this chapter, we're immersing ourselves in the setup and configuration of the
infrastructure/data
module. This module is responsible for implementing the repository contracts defined in the
business-repository
module using Quarkus Hibernate Reactive Panache, and it also integrates with a MySQL database.
Powering Data Management with Panache and MySQL
The
infrastructure/data
module lies at the heart of data management, seamlessly connecting our core business logic with the persistence layer. By combining the efficiency of Quarkus Hibernate Reactive Panache and the reliability of MySQL, this module delivers a robust and responsive data management solution.
Dependency Setup
By depending on the
domain-model
module, we establish a direct relationship between our data repository contracts and the core domain models they interact with. This ensures that our repository interfaces align seamlessly with the underlying data structure.
Before we proceed, let's explore the dependencies that the
infrastructure/data
module relies on:
-
domain-model
: This module provides core domain models that the repository implementations interact with. -
business-repository
: The repository contracts defined in this module drive the implementations in theinfrastructure/data
module. -
io.quarkus:quarkus-panache-common
: This Quarkus extension offers the common utilities for Panache entities. -
io.quarkus:quarkus-hibernate-reactive-panache
: The Quarkus extension for Hibernate Reactive Panache, enabling reactive database operations. -
io.quarkus:quarkus-reactive-mysql-client
: Quarkus extension for reactive MySQL database operations.
dependencies { implementation project(':domain-model') implementation project(':business-repository') implementation 'io.quarkus:quarkus-panache-common' implementation 'io.quarkus:quarkus-hibernate-reactive-panache' implementation 'io.quarkus:quarkus-reactive-mysql-client' }
Integrating with MySQL
The integration with MySQL is facilitated by the Quarkus extension
io.quarkus:quarkus-reactive-mysql-client
. By using this extension, our repository implementations can seamlessly interact with a MySQL database using reactive patterns.
For detailed guidance on setting up MySQL with Quarkus, refer to the Quarkus Guide on MySQL Reactive Datasource.
Repository Implementations
The core responsibility of the
infrastructure/data
module is to implement the repository contracts defined in the
business-repository
module. Using Panache's reactive database repository classes, we encapsulate the logic for CRUD operations, translating between domain models and JPA entities.
For a comprehensive walkthrough of using Hibernate Reactive Panache, check out the Quarkus Guide on Hibernate Reactive.
In this segment, we've embarked on a journey through the configuration of the
infrastructure/data
module, exploring its dependency setup and integration with Quarkus Hibernate Reactive Panache and MySQL. By combining the power of reactive database operations with efficient data management, we pave the way for a resilient and responsive application.
You can find the code of this section in the branch: steps/5-configuring-infrastructure-data
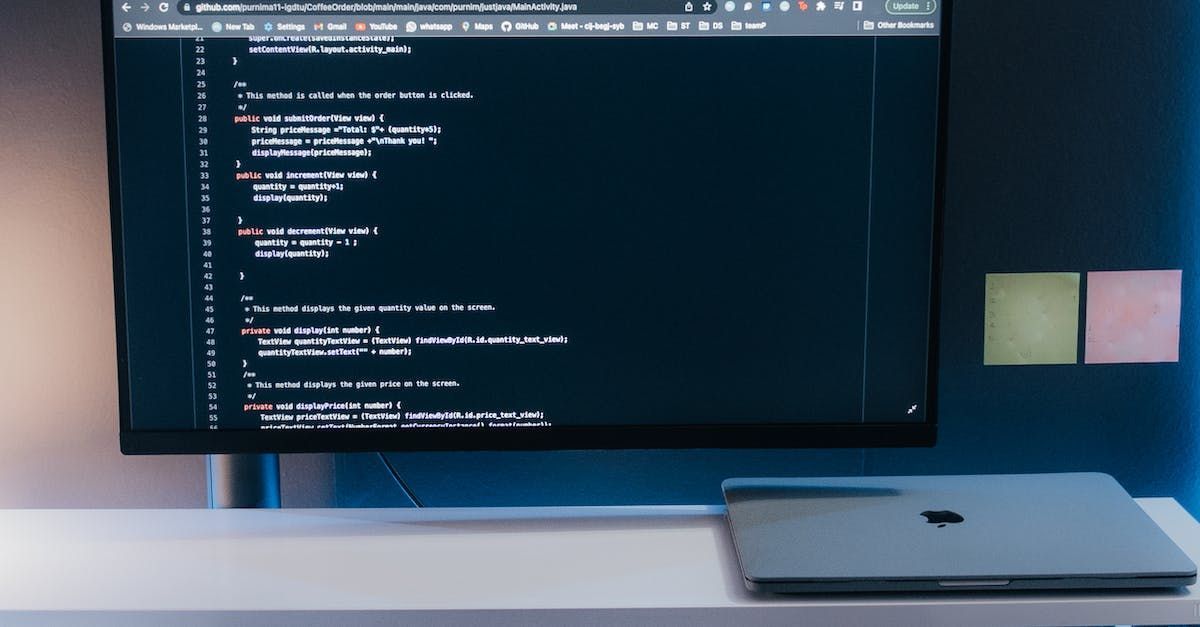
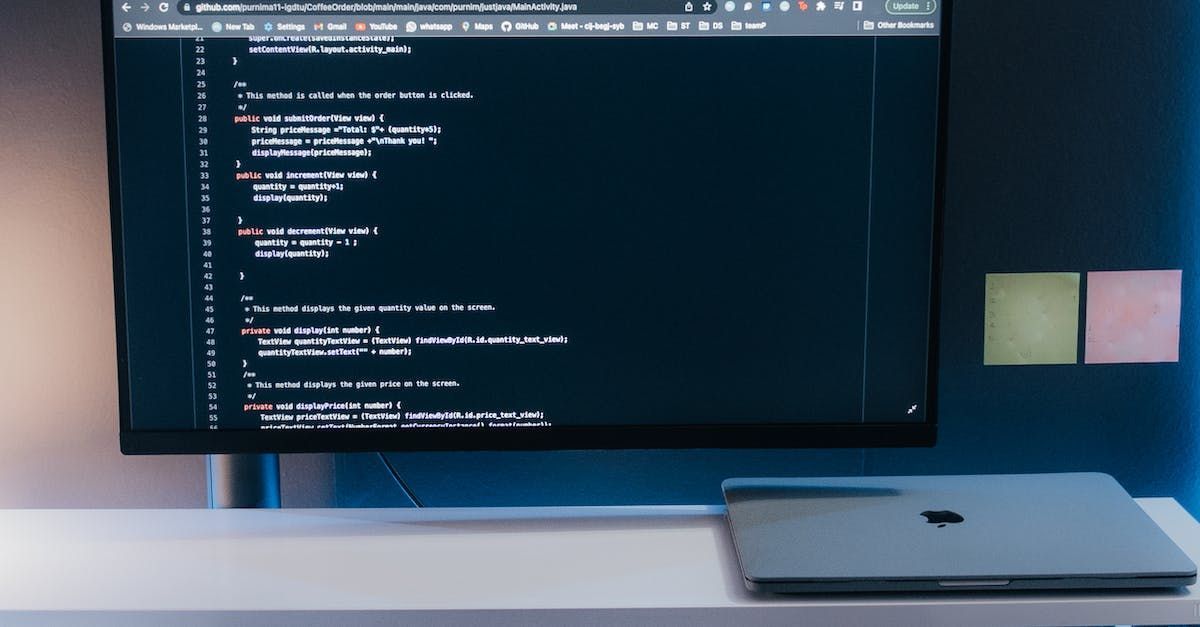